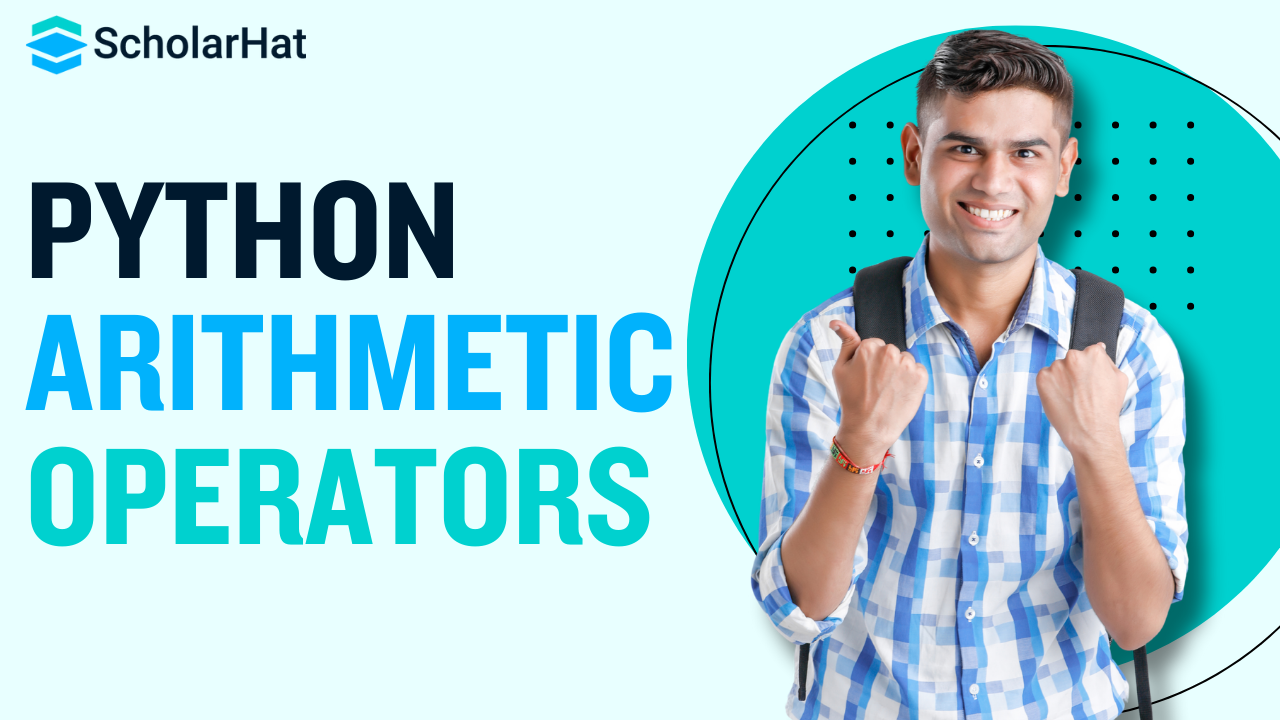
Python Arithmetic Operators: A Comprehensive Guide
Introduction to Python Arithmetic Operators
If you’re delving into the world of Python programming, understanding arithmetic operators is paramount. These operators allow you to perform various mathematical computations and manipulations within your code. Let’s take a deep dive into the world of Python arithmetic operators and uncover their functionalities.
Understanding Python Arithmetic Operators
In Python, arithmetic operators are symbols used to perform mathematical operations on numeric operands. The primary operators include addition (+), subtraction (-), multiplication (*), division (/), modulus (%), exponentiation (**), and floor division (//).
Addition and Subtraction
The addition operator (+) in Python is used to add two numbers together. For instance, in the expression a + b, the operator ‘+’ adds the values stored in variables a and b. Conversely, the subtraction operator (-) subtracts the second operand from the first.
Example:
python
Copy code
x = 10
y = 5
result_addition = x + y # Result will be 15
result_subtraction = x – y # Result will be 5
Multiplication and Division
The multiplication (*) operator is used to multiply two numbers, while the division (/) operator divides the first operand by the second. It’s crucial to note that Python Tutorial supports division in different ways, including floating-point division and integer division.
Example:
python
Copy code
p = 8
q = 4
result_multiplication = p * q # Result will be 32
result_division = p / q # Result will be 2.0
Modulus, Exponentiation, and Floor Division
The modulus (%) operator returns the remainder of the division between two numbers. Exponentiation (**), on the other hand, raises the first operand to the power of the second operand. Lastly, floor division (//) performs division where the result is rounded down to the nearest whole number.
Example:
python
Copy code
m = 20
n = 3
result_modulus = m % n # Result will be 2
result_exponentiation = m ** n # Result will be 8000
result_floor_division = m // n # Result will be 6
Applications of Python Arithmetic Operators
Arithmetic operators find extensive usage in various programming scenarios. From simple calculations to complex mathematical operations, these operators form the backbone of numerical manipulation in Python.
Using Arithmetic Operators in Control Structures
Python’s arithmetic operators are not confined to basic calculations. They play a pivotal role within control structures like loops and conditional statements. They enable dynamic computations and decision-making processes within the code.
Arithmetic Operators in Data Manipulation
In data analysis and manipulation, arithmetic operators prove invaluable. Whether it’s performing calculations on arrays, manipulating matrices, or processing numerical data, these operators facilitate efficient data handling and transformation.
Python Arithmetic Operators in Real-World Applications
Python’s arithmetic operators extend beyond theoretical understanding; they are fundamental in real-world applications. From financial calculations in banking systems to scientific computations in research laboratories, Python’s versatility in handling numerical operations is unparalleled.
Python Arithmetic Operators in Financial Systems
In financial systems, arithmetic operators play a crucial role in computing interest rates, handling transactions, and performing complex calculations for investment portfolios. These operators ensure accurate financial modeling and analysis.
Scientific Computation using Python Arithmetic Operators
Scientific computations demand precise numerical handling. Python’s arithmetic operators are extensively employed in scientific research for simulations, data analysis, and complex mathematical modeling, enabling scientists to derive accurate conclusions from experimental data.
Conclusion
In conclusion, understanding Python arithmetic operators is indispensable for anyone venturing into programming. These operators, from addition to floor division, empower programmers to perform diverse mathematical operations with ease and precision. Whether you’re a novice or an experienced coder, harnessing the potential of these operators enhances your capability to craft efficient and robust Python programs.
Python’s arithmetic operators serve as the cornerstone of numerical manipulation, offering a vast array of functionalities for diverse applications. Embrace these operators, delve into their nuances, and unlock the true potential of Python programming. Mastering them will undoubtedly elevate your coding prowess and open doors to a world of endless possibilities.
Remember, Python arithmetic operators are not mere symbols; they are the tools that transform mathematical abstractions into tangible solutions.
So, dive in, explore, and let Python arithmetic operators amplify your programming journey!
By – ScholaHat