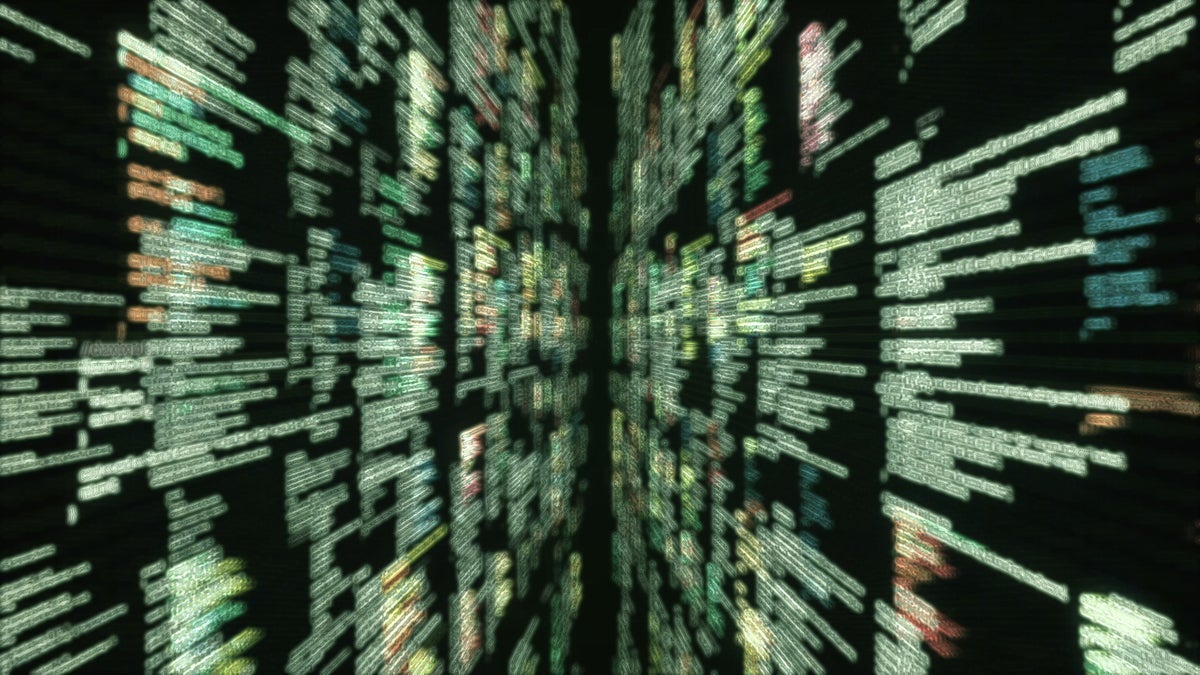
Bash scripting ideas that can preserve time on Linux
[ad_1]
Committing regime and even hardly ever required jobs to scripts is nearly constantly a big earn since you don’t have to reinvent the method to obtaining do the job performed every time it’s needed, and you help you save a great deal of time on problems you manage normally.
Below are some recommendations for composing bash scripts and guaranteeing that they’ll be uncomplicated to use, quick to update/ and difficult to misuse.
Responses
A person crucial detail to do when you might be planning a script on Linux is to insert reviews – specially for instructions that could be a minimal sophisticated. If you never operate a script extremely typically, comments can assist make sure that you immediately grasp anything that it’s doing. If another person else has to use your scripts, the feedback can make it a whole lot easier for them to know what to be expecting. So, constantly increase reviews. Even you may well appreciate them! You do not require to comment just about every line, just every single important team of instructions. Here is a easy illustration.
#!/bin/bash # purpose to switch spaces in offered text with underscores replspaces () echo $@ # functionality to clear away spaces from the presented text dropspaces () echo $@ # commands to connect with the capabilities outlined earlier mentioned replspaces "Good day Globe" replspaces `date` dropspaces "Howdy Planet" dropspaces `date`
The script higher than defines two functions: one to convert areas into underscores and 1 to get rid of areas completely in no matter what string is provided at the prompt. The opinions say plenty of to make clear this.
Utilizing capabilities
As observed in the script above, functions can appear in useful. This is especially legitimate when a one script will run the capabilities a lot of moments. The scripts will be shorter and will be simpler to have an understanding of.
Verifying arguments
Make a habit of getting your scripts verify that the proper arguments have been furnished by whomever operates them. You can examine the number of arguments, but you can also verify that the responses are valid. For illustration, if you prompt for the name of a file, verify that the file furnished in fact exists right before running instructions that try to use it. If it won’t exist, react with an error and exit.
The script under checks to ensure that two arguments have been furnished as arguments to the script. If not, it prompts for the data desired.
#!/bin/bash if [ $# -lt 2 ] then echo "Usage: $ strains filename" exit 1 else numlines=$1 filename=$2 fi …
The script below prompts for a filename and then checks to see if the file exists.
#!/bin/bash # get title of file to be study echo -n "filename> " study $filename # look at that file exists or exit if [ ! -f $filename ] then echo "No these file: $filename" exit fi
If necessary, you can also test whether the file is writable or readable. The variation of the script under does both of those.
#!/bin/bash # get name of file to be examine echo -n "filename> " read filename # look at that file exists or exit if [ ! -f $filename ] then echo "No such file: $filename" exit else # figure out if file is readable if [ ! -r $filename ] then echo "$filename is not writable" exit fi
# establish if file is writable if [ ! -w $filename ] then echo "$filename is not readable" exit fi fi
Observe: The -r $filename check asks regardless of whether the file is readable. The ! -r $filename check asks no matter if it is not readable.
Exiting on faults
You need to just about always exit when an mistake is encountered when a script is operate. Describe with an error information what went erroneous. The set -o errexit will trigger a script to exit irrespective of what kind of error is encountered.
#!/bin/bash established -o errexit # exit on ANY error tail NoSuchFile # tries to display bottom strains in NON-EXISTENT file echo -n "Enter text to be appended> " go through txt echo $txt >> NoSuchFile
The script previously mentioned will exit if the “NoSuchFile” file does not exist. An mistake will be displayed as nicely.
To exit when a variable hasn’t been assigned a benefit (i.e., doesn’t exist), use the nounset possibility as revealed in the script down below. A established -u command is effective the similar.
#!/bin/bash # exit script if an unset variable is made use of established -o nounset # welcome user with a greeting echo $greeting echo -n "Enter title of file to be evaluated: " examine filename
If you operate the script as is, you are going to operate into the challenge proven underneath and by no means be prompted for the file to be processed:
$ ck_nounset
./ck_nounset: line 7: greeting: unbound variable
Working with rates
In a lot of situations, it does not make any difference if you use solitary quotes, double offers, or no quotations at all. Placing quotations around a variable name in an if assertion suggests that you won’t operate into an mistake if the variable is not outlined. The shell will under no circumstances close up seeking to assess the invalid command if [ = 2 ]. The command if [ “” = 2 ] is valid.
if [ "$var" = 2 ]
Putting prices all around arguments with far more than a single string is critical. You’d operate into complications with the script below if the consumer answering the excellent/poor concern entered “really excellent” instead of just “great” or “negative”.
#!/bin/bash echo -n "Did you uncover the argument to be excellent or poor?> " browse ans if [ $ans = good ] then echo "Thank you for your feed-back" else echo -n "What did not you like?> " read ans echo $ans >> poor_opinions fi
Instead, you could use a line like this to do the comparison. This would allow both “very good” or “very great” as a response.
if [[ "$ans" = *"good" ]] then
Utilizing = or ==
Most of the time it makes no variance regardless of whether you use = or == to evaluate strings. On the other hand, some shells (e.g., dash) don’t work with ==, so save oneself the keystrokes and stick with = if you might be not absolutely sure.
File permissions
Make confident your scripts can be run by any one who should be equipped to use them. A chmod -ug+rx filename command would give you (presumably the file operator) and anybody in the group affiliated with the file the potential to examine and run the script.
Exam your scripts
Do not overlook to invest some time testing to make sure your scripts do precisely what you intend. Screening can consist of items like failing to answer to the script’s prompts to see how it reacts.
Wrap-Up
Theres more suggestions about setting up trustworthy and very easily maintainable scripts together with studying to script on Linux employing bash, lots of strategies to loop working with bash, and how to loop endlessly in bash.
Copyright © 2023 IDG Communications, Inc.
[ad_2]
Supply backlink